A storage Classe represents visibility as well as the location of a variable. It distinguishes what part of code we can access a variable. A storage class is used to describe the complying with points:
- The variable range.
- The area where the variable will be kept.
- The initialized value of a variable.
- A lifetime of a variable.
- Who can access a variable?
Therefore a storage class is utilized to stand for the details regarding a variable.
NOTE: A variable is not only related to a data kind, its worth however likewise a storage class.
There is a total of four kinds of standard storage classes.
C Language has four storage classes
- Auto Storage class
- Register Storage class
- Static storage class
- External storage class
1. Auto storage class
The variables defined by making use of auto storage class are called local variables. Auto means an automated storage class. A variable is in the auto storage space course by default if it is not clearly defined.
The range of an auto variable is restricted with a particular block only. As soon as the control goes out of the block, the gain access to is ruined. This means only the block in which the auto variable is proclaimed can access it.
A keyword auto is utilized to define an auto storage space course. By default, an auto variable includes garbage worth.
Example, auto int age;
Program in C for Auto storage class understanding
[c]
#include <stdio.h>
#include<conio.h>
void main( )
{
auto int j = 1;
{
auto int j= 2;
{
auto int j = 3;
printf ( ” %d “, j);
}
printf ( “%d “,j);
}
printf( “%d\n”, j);
getch();
}
[/c]
Output:-
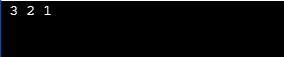
2. Register Storage class
You can make use of the register storage space course when you intend to keep neighborhood variables within features or blocks in CPU signs up instead of RAM to have quick access to these variables. As an example, “counters” are a great prospect to be stored in the register.
Example: register int age;
The keyword register is made use of to state a register storage course. The variables stated using register storage space class have life-span throughout the program.
It resembles the automobile storage course. The variable is restricted to the particular block. The only distinction is that the variables declared utilizing register storage space class are kept inside CPU signs up instead of a memory. The register has much faster accessibility than that of the main memory.
The variables stated utilizing register storage class has no default worth. These variables are usually stated at the beginning of a program.
Program in C for register storage class understanding
[c]
#include <stdio.h>
#include<conio.h>
#include <stdio.h>
void main()
{
{
register int weight;
int *ptr=&weight ;
}
getch();
}
[/c]
The Static variables are utilized within function/ file as neighborhood fixed variables. They can also be used as a global variable
3. Static storage class
A static local variable is a neighborhood variable that maintains as well as saves its value between function calls or block and continues to be noticeable only to the function or block in which it is defined.
Static international variables are global variables noticeable just to the documents in which it is declared.
Example: static int count = 10;
Keep in mind that the static variable has a default initial value zero and is initialized only once in its lifetime.
Program in C to understand static storage class
[c]
#include <stdio.h>
#include<conio.h>
void display();
int main()
{
display();
display();
}
void display()
{
static int a = 2;
a += 6;
printf(“%d “,a);
getch();
}
[/c]
Output

4. External storage class
The extern storage space class is made use of to give a recommendation of a global variable that shows up to ALL the program documents. When you utilize ‘extern’, the variable can not be initialized nevertheless, it directs the variable name at a storage place that has actually been previously defined.
When you have multiple data, as well as you, define a global variable or feature, which will certainly also be utilized in various other documents, then extern will be made use of in another data to supply the recommendation of specified variable or feature. Just for understanding, extern is made use of to proclaim an international variable or feature in additional data.
The extern modifier is most generally used when there are 2 or more data sharing the same international variables or functions as discussed listed below.
Program in C to understand External storage classes
First File: main.c
[c]
#include <stdio.h>
#include<conio.h>
void display();
int main()
{
display();
display();
}
void display()
{
static int a = 2;
a += 6;
printf(“%d “,a);
getch();
}
[/c]
Second File: support.c
[c]
#include <stdio.h>
extern int count;
void write_extern(void) {
printf(“count is %d\n”, count);
}
[/c]
Here, extern is being used to declare count in the second file, where as it has its definition in the first file, main.c. Now, compile these two files as follows
$gcc main.c support.c
It will produce the executable program a.out. When this program is executed, it produces the following result −
count is 5