- C program to print all natural numbers from 1 to n using while loop.
- C program to print all even numbers between 1 to 100 using while loop.
- C program to print all odd number between 1 to 100 using while loop.
- C program to print sum of all even numbers between 1 to n using while loop.
- C program to print sum of all odd numbers between 1 to n using while loop.
- C program to print multiplication/table of any number using while loop.
- C program to count the digits of a given number using while loop.
- C program to print the sum of digits of a given number using while loop.
- C program to print all natural numbers in reverse order using while loop.
- C program to check whether a given number is Prime or not using while loop.
- C program to print all Prime numbers between 1 to n using while loop.
- C program to find sum of all prime numbers between 1 to n using while loop.
- C program to check a given number is Armstrong or not using while loop.
- C program to print all Armstrong numbers between 1 to n using while loop.
- C program to check a given number is Perfect or not using while loop.
- C program to check all Perfect numbers between 1 to n using while loop.
- C program to check a given number is Strong number or not using while loop.
- C program to print all Strong numbers between 1 to n using while loop.
- Write a program in C to swap first and last digit of number using while loop.
- C Program to swap values using third variable using while loop.
- C Program to swap values without using third variable using while loop.
- C program to calculate power of a number without using pow method using while loop.
- C program to find power of a number without using pow method using while loop.
- C program to calculate power of a number using pow method using while loop.
- C Program to check number is palindrome or not using while loop.
- C Program to print Fibonacci series of a given number using while loop.
- C Program to print Fibonacci series of a given number using recursion using while loop.
- C Program to print the frequency of digits in given number using while loop.
- Write a program in C to print ASCII character with values using while loop.
- C program to print all alphabets from a to z using while loop.
- C program to print all uppercase alphabets using while loop.
- C program to print all lowercase alphabets using while loop.
- C program to print all factors of a number using while loop.
- C program to print HCF (GCD) of two numbers using while loop.
- C program to print LCM of two numbers using while loop.
- Write a C program to find all prime factors of a number using while loop.
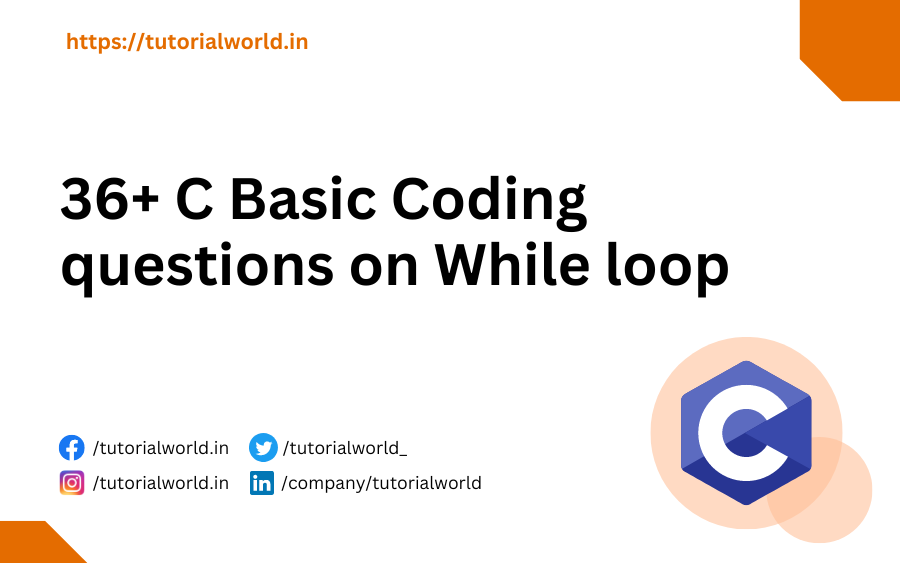