In this tutorial, We will learn writing to reverse the array elements. To reverse the array element in java there are multiple ways to achieve it.
Our program will first take the input of array size and then the elements of the array. And return the reverse of the input array.
For Example:
For array arr=[1,2,3,4,5,6]
Output should be [6,5,4,3,2,1]
Program 1: Using temp array reverse the array element
- In this array reversal program we have created a temp array and temp variable.
- Here we are iterating the array element from last to first and storing the elements in temp array.
- Now to print array in reverse order, we will be iteraing the temp array, Output will be print in reverse order.
import java.util.*;
class Main{
public static void main(String ...a){
Scanner sc = new Scanner(System.in);
System.out.println("Enter the size of array: ");
int n = sc.nextInt();
int arr[] = new int[n];
int temp[] = new int[n];
int tempVar=n;
System.out.println("Enter " +(n)+ " array elements: ");
for(int i=0; i<n; i++) {
arr[i] = sc.nextInt();
}
for (int i = 0; i < n; i++) {
temp[tempVar - 1] = arr[i];
tempVar = tempVar - 1;
}
System.out.println("Array after Reverse is : ");
for (int i = 0; i < n; i++) {
System.out.println(temp[i]);
}
}
}
Output
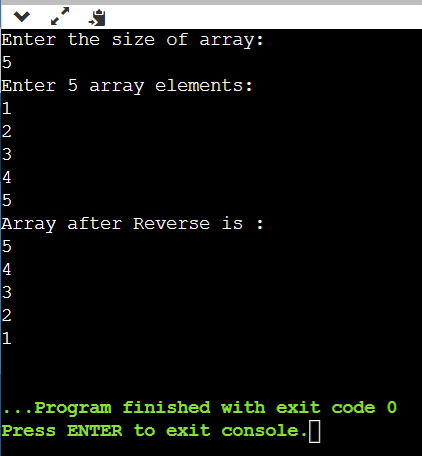
Program 2: Using Collections.reverse() method to reverse array element
- This program is something different from above because here we are using the existing function to reverse the array.
- Collections.reverse() is a inbuild method inside java.util package.
- Collections.reverse() will take array list as an input and then reverse the array.
- Here we don’t need any extra temp variable or array. It reverse the original array.
- After reversing we are printing the output using System.out.println.
import java.util.*;
class Main{
public static void main(String ...a){
Scanner sc = new Scanner(System.in);
System.out.println("Enter the size of array: ");
int n = sc.nextInt();
Integer arr[] = new Integer[n];
System.out.println("Enter " +(n)+ " array elements: ");
for(int i=0; i<n; i++) {
arr[i] = sc.nextInt();
}
System.out.println("Array After Reversal : ");
Collections.reverse(Arrays.asList(arr));
System.out.println(Arrays.asList(arr));
}
}
Output
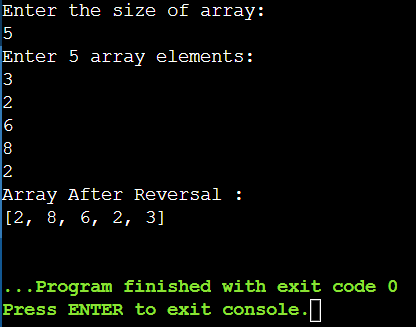