In this tutorial, we will learn how Comparator and Comparable in Java, works with the help of examples. Both comparator and comparable are used to sort the list of elements.
Comparable Interface
Comparable is a public interface that provides a single sorting sequence and it is present in java.lang package. Basically it follows the ASCII sequence which is a natural ordering. Natural ordering means the number will be sort in 1,2,3 and alphabets will be sort like ‘a’,’b’,’c’.
Comparable Interface uses the sorting technique of JVM by default. It has a public compareTo() method for sorting the elements. compareTo() method takes one object as an argument.
Examples of Comparable:
[cc lang=”java” tab_size=”2″ lines=”80″]
import java.util.*;
import java.io.*;
class Student implements Comparable
int rollno;
int age;
String name;
Student(int rollno,int age,String name){
this.rollno=rollno;
this.age=age;
this.name=name;
}
public int compareTo(Student s){
if(age==s.age)
return 0;
else if(age>s.age)
return 1;
else
return -1;
}
}
public class Main{
public static void main(String args[]){
ArrayList
arr.add(new Student(101,20,”Prakash”));
arr.add(new Student(102,22,”Saurav”));
arr.add(new Student(103,19,”Raj”));
Collections.sort(arr);
for(Student s:arr){
System.out.println(s.rollno+” “+s.age+” “+s.name);
}
}
}
[/cc]
Output

Comparator Interface
Comparator is a public interface that provides multiple sorting sequence and it is present in java.util package. It has a public compare() method for sorting the elements. compare() method takes two objects as an argument.
Example of Comparator:
[cc lang=”java” tab_size=”2″ lines=”80″]
import java.util.*;
import java.io.*;
class Student{
int rollno;
String name;
int age;
Student(int rollno,String name,int age){
this.rollno=rollno;
this.name=name;
this.age=age;
}
}
class AgeComparator implements Comparator
public int compare(Student s1,Student s2){
if(s1.age==s2.age)
return 0;
else if(s1.age>s2.age)
return 1;
else
return -1;
}
}
class NameComparator implements Comparator
public int compare(Student s1,Student s2){
return s1.name.compareTo(s2.name);
}
}
class Main{
public static void main(String args[]){
ArrayList
arr.add(new Student(101,”Prakash”,20));
arr.add(new Student(103,”Rohan”,25));
arr.add(new Student(102,”suresh”,21));
System.out.println(“Sorting by Name”);
Collections.sort(arr,new NameComparator());
for(Student st: arr){
System.out.println(st.rollno+” “+st.name+” “+st.age);
}
System.out.println(“sorting by Age”);
Collections.sort(arr,new AgeComparator());
for(Student st: arr){
System.out.println(st.rollno+” “+st.name+” “+st.age);
}
}
}
[/cc]
Output
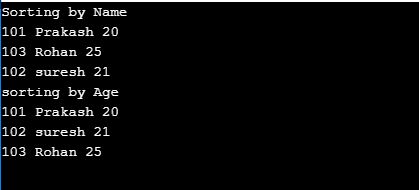
Difference Between Comparator and Comparable in Java
Comparable:
- Comparable can sort the elements only on the basis of a single element in one go. Single element means if we have student data then we can sort only on the basis of either using id, name, or price.
- Comparable have a compareTo() method for sorting the elements.
- compareTo() method takes only one object as a parameter.
- Using Collection.sort(List) method we can sort the list of elements.
- Comparable is available in java.lang package.
Comparator:
- A comparator can sort the elements on the basis of multiple elements in one go.
- Comparable have compare() method for sorting the elements.
- compare() method takes two objects as a parameter.
- Using Collection.sort(List, Comparator) method we can sort the list of elements.
- Comparable is available in java.util package.