Java Enhanced For Loop was introduced in Java 5. It is also know as for each loop. It is a simpler way to iterate through all the elements of a Collection and arrays.
Enhanced for
loops are simple but inflexible. This for loop widely used when we don’t know the last element of the array or collections. When we want to step through the elements of the array in first-to-last order, and you do not need to know the index of the current element, We can use it.
Break and Continue statement is also used in this enhanced for loop to control the flow statement.
Java Enhanced For Loop Syntax or for each syntax
[java]
for(dataType item : array) {
…
}
[/java]
In the above for each syntax,
- array – this is an array or a collection
- item – this is a variable in which each item will be assign while iteration
- dataType – data type represents the the array/collection datatype
Java Enhanced For Loop Examples
Example 1: Iterate and print the integer array using for loop
[java]
class Main {
public static void main(String[] args) {
int[] items = {1, 2, 3, 4};
for (int item: items) {
System.out.println(item);
}
}
}
[/java]
Output
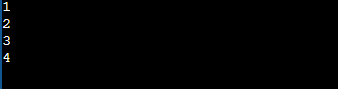
For Loop Vs Enhanced For Loop With Examples
For Loop Example
Here using For Loop we are iterating the array elements. This for loop is little different syntax wise. Functionality wise both are same because both have same work to do I mean iterating the array elements or collection elements.
[java]
class Main {
public static void main(String[] args) {
int[] items = {2, 4, 6, 8};
for (int i=0; i<items.length ; i++) {
System.out.println(items[i]);
}
}
}
[/java]
Output
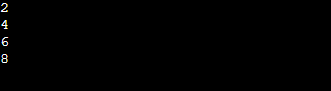
Enhanced For Loop Example
[java]
class Main {
public static void main(String[] args) {
int[] items = {2, 4, 6, 8};
for (int item : items) {
System.out.println(item);
}
}
}
[/java]
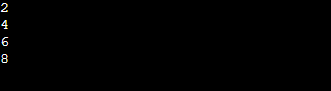