In this tutorial, you’ll learn how to write program in check if the given year is a leap year or not. Or We can say leap year program in Java. To write the leap year program in Java we will be using the conditional statement to perform check which is if else statement.
What is Leap Year?
A leap year is a year that is divisible by 4. Century years which is ending with 00 is not coming in this divisible by 4 category. The century year is a leap year only if it is perfectly divisible by 400.
This is some conditions for a leap year
A year is a leap year if
- Year is evenly divisible by 100.
- If year is divisible by 100, then it should also be divisible by 400.
- For non century years, Year should evenly divisible by 4 are leap years.
Programs
Program 1: Leap year program in Java
[java]
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
int year;
System.out.println(“Enter an Year : “);
Scanner sc = new Scanner(System.in);
year = sc.nextInt();
boolean leap = false;
if (year % 4 == 0) {
if (year % 100 == 0) {
if (year % 400 == 0)
leap = true;
else
leap = false;
}
else
leap = true;
}
else
leap = false;
if (leap)
System.out.println(“Given year ” + year + ” is a leap year.”);
else
System.out.println(“Given year ” + year + ” is not a leap year.”);
}
}
[/java]
Output
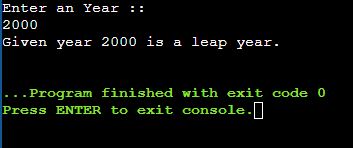
Program 2: Leap Year Program in java
[java]
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
int year;
System.out.println(“Enter an Year : “);
Scanner sc = new Scanner(System.in);
year = sc.nextInt();
if (((year % 4 == 0) && (year % 100!= 0)) || (year%400 == 0))
System.out.println(“Given year ” + year + ” is a leap year.”);
else
System.out.println(“Given year ” + year + ” is not a leap year.”);
}
}
[/java]
Output
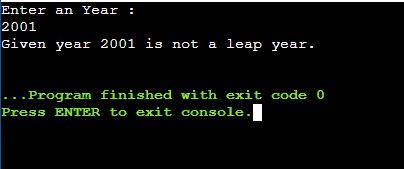