In this program, you’ll learn writing the Swapping of two numbers in java. We will see different techniques to swap two numbers in Java.
- First approach of swapping will be by using the temporary variable
- The second approach will be without using the any temporary variables.
Swapping of number
Suppose we have two numbers a and b, Now in swapping we will exchange the value which means a value will be assign to b and b value will assign to a.
Examples:
Input : a = 40, b = 20;
Output : b = 20, a = 40
Input : a = 300, b = 100
Output : b = 100, a = 300
Program 1: Swap two numbers using temporary variable
[java]
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
int a,b,temp;
System.out.println(“Enter the number for ‘a’: “);
Scanner sc = new Scanner(System.in);
a = sc.nextInt();
System.out.println(“Enter the number for ‘b’: “);
b = sc.nextInt();
temp = a;
a = b;
b = temp;
System.out.println(“a = “+ a +” b = “+ b );
}
}
[/java]
Output
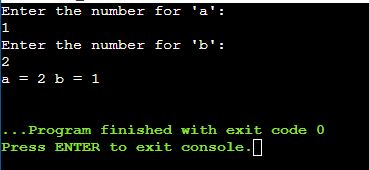
Explanations
In the above program we have taken two variables a and b. And we are taking input from the users. Now our target is to swap this value means value given to a should assign to b and the value of b should assign to a.
- Value of variable a will be stored in the temporary variable temp.
- Then the value of b will be sorted in variable a.
- And, finally value of temp variable is stored in b.
- This is the completes process of swapping of the two variables in java using third temporary variable.
Example 2: Swap two numbers without using temporary variable
[java]
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
int a,b,temp;
System.out.println(“Enter the number for ‘a’: “);
Scanner sc = new Scanner(System.in);
a = sc.nextInt();
System.out.println(“Enter the number for ‘b’: “);
b = sc.nextInt();
a = a – b;
b = a + b;
a = b – a;
System.out.println(“a = “+ a +” b = “+ b );
}
}
[/java]
Output
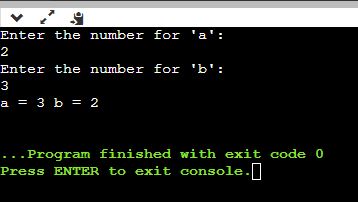
Explanation
In the above program we are not using any third or temporary variable what we have done in previous program. Here we are using simple mathematics to swap the numbers.
This is all about the swapping of two numbers in java using temp variable and without using the temp variable.