In this tutorial, you will learn to write a program in java to count the duplicate characters available in a string. In other words, we can say if a character is occurred more than one time then count them.
Example:
Suppose the user has given input like aabbcddde
then the output should be 3 because only three characters a, b, and d occurred multiple times which is a duplicate.
Program 1: Java Program to count duplicate charcter in String
[java]
import java.util.*;
public class Main
{
public static void main(String[] args) {
Scanner sc= new Scanner(System.in);
System.out.print(“Please enter a string: “);
String str= sc.nextLine();
int count, duplicateCount = 0;
char strArr[] = str.toCharArray();
System.out.println(“Count of the Duplicate characters in string: “);
for(int i = 0; i < strArr.length; i++) {
count = 1;
for(int j = i+1; j <strArr.length; j++) {
if(strArr[i] == strArr[j] && strArr[i] != ‘ ‘) {
count++;
strArr[j] = ‘0’;
}
}
if(count > 1 && strArr[i] != ‘0’)
duplicateCount++ ;
}
System.out.println( “Total duplicate Character = “+duplicateCount);
}
}
[/java]
Output
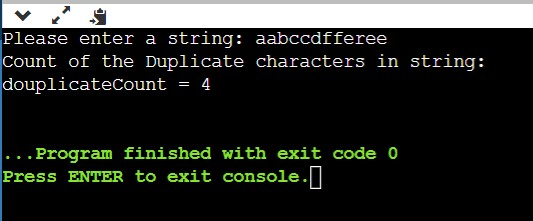