To insert an element at the end of an array, first we have to iterate the array till the end and then add an element at the end of the array. We will be using for loop to iterate the array.
For example:
Suppose we have an array arr = [2, 3, 4, 6, 7]
After inserting 8 at end of the array our array arr will become arr = [2, 3, 4, 6, 7, 8]
Program 1: Program in java to Insert element at the end of array.
- The below program is all about inserting the element at the end of an array.
- Here we are taking the array size and input elements from the users.
- While taking the input size, we are increasing the array size by one so that we can insert a new element at the end of the array.
- After inserting, using for loop we iterate and print the value.
import java.util.*;
public class Main
{
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Java Program to insert element at end of Array");
System.out.print("Enter the size of array: ");
int size = sc.nextInt();
int arr[] = new int[size+1];
for(int i=0; i<size; i++) {
System.out.print("Please give value for index "+ i +" : ");
arr[i] = sc.nextInt();
}
System.out.print("Enter the element to insert at end: ");
arr[size] = sc.nextInt();
System.out.println("Array After Inserting "+ arr[size] +" at end");
for(int i=0; i<size+1; i++)
{
System.out.println(arr[i]);
}
}
}
Output
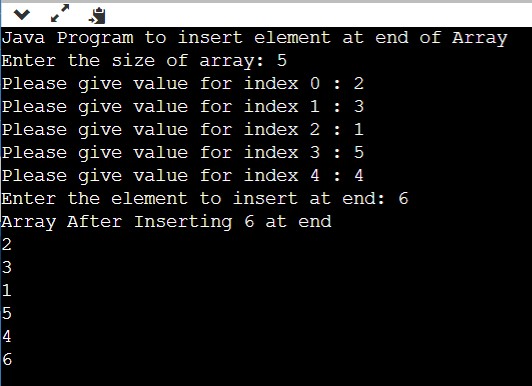
Program 2: Inserting an element at the end of ArrayList Java
- Here We have taken the ArrayList to insert the element at the end of the Array.
- ArrayList maintains the insertion order.
- And Also we don’t have to increase the size, it will grow dynamically.
import java.util.*;
class Main{
public static void main(String[] args) {
List<String> list = new ArrayList<String>();
list.add("Ram");
list.add("Soham");
list.add("Dinesh");
Scanner sc = new Scanner(System.in);
System.out.println("Please give string to insert: ");
String newElement = sc.nextLine();
list.add(newElement);
System.out.println("ArrayList after inserting element at end: ");
list.stream().forEach(System.out::println);
}
}
Output
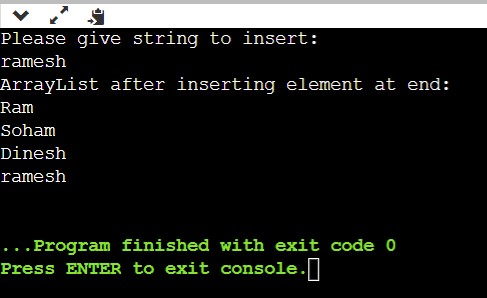