To insert an element at the beginning of an array, we have to shift all element to the right. After shifting to the right, the zero index will become free then insert a new element at the zero position.
For Example:
Suppose we have an array arr=[2, 4, 1, 6, 3]
After inserting 7 at beginning of an array, arr will become arr=[7, 2, 4, 1, 6, 3]
Java program to insert element at the beginning in an array
import java.util.*;
public class Main
{
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Java Program to insert element at beginning of Array");
System.out.print("Enter the size of array: ");
int size = sc.nextInt();
int arr[] = new int[size+1];
for(int i=0; i<size; i++) {
System.out.print("Please give value for index "+ i +" : ");
arr[i] = sc.nextInt();
}
System.out.print("Enter the element to insert at first: ");
int newElement = sc.nextInt();
for(int i=size; i>0; i--)
{
arr[i]=arr[i-1];
}
arr[0]=newElement;
System.out.println("Array After Inserting "+ arr[0] +" at beginning : ");
for(int i=0; i<=size; i++) {
System.out.print(arr[i]);
}
}
}
Output
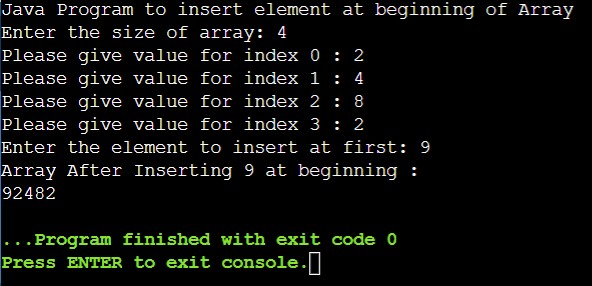