To insert an element in the array at a given location using java first we have to perform the right shift by one so that we can insert the element at the given location.
Here we are treating location and index differently. Location means an exact place which we can consider starting from 1. But the array index is something that starts from 0.
For Example:
Suppose we have an array arr = [2,3,5,6,8]
now we have to insert 4 at location 2
So new array will become arr = [2,4,3,5,6,8]
Program 1: Program to insert element in array at a given location in Java
- As we know that in array iteration not required to reach an index because we have an index number. And using index numbers we can reach that particular index.
- Now to insert the element at a given location, we will perform the right shift on the array element till the location.
- It will free the location index where we have to insert the element.
- Here we are considering location and index different because for location 2 array index will be one. Because the array index starts with 0 but location we will consider starting from 1.
- After shifting the elements right to the location, we can insert elements easily.
import java.util.*;
public class Main
{
public static void main(String[] args) {
int loc;
Scanner sc = new Scanner(System.in);
System.out.println("Java Program to insert element at given location of Array");
System.out.print("Enter the size of array: ");
int size = sc.nextInt();
int arr[] = new int[size+1];
for(int i=0; i<size; i++) {
System.out.print("Please give value for index "+ i +" : ");
arr[i] = sc.nextInt();
}
System.out.print("Enter the location where you want to insert:");
loc = sc.nextInt();
for (int i = size-1; i >= loc-1; i--){
arr[i+1] = arr[i];
}
System.out.print("Enter the element to insert at location "+loc+" : ");
arr[loc-1] = sc.nextInt();
System.out.println("Array After Inserting "+ arr[loc-1] +" at location "+loc+" : ");
for(int i=0; i<size+1; i++)
{
System.out.print(arr[i]);
}
}
}
Output
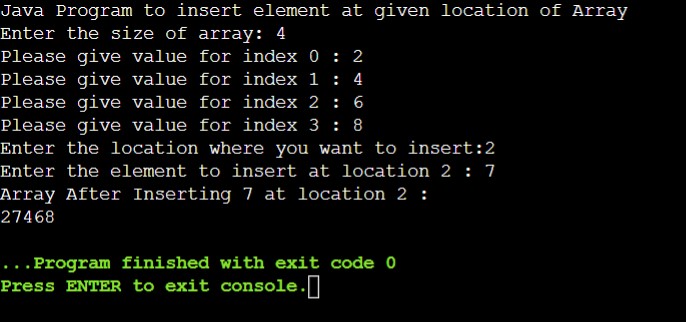