In this tutorial, we will learn to write a java program to find the maximum and minimum elements in an array.
For example,
Input: arr[] = [1, 7, 5, 2, 9, 8]
Output:
Minimum array element : 1
Maximum array element : 9
Program 1 : Find maximum and minimum in array java program
- In the below program we are taking array size and array elements from the users as an input to find maximum minimum in array java.
- Now we have two variable max and min to store the maximum and minimum value from the array.
- Here we are iterating the each elements and comparing the previous value stored in max and min.
- If greater value founded then replace it with the old value of max and if smaller value found replace it with the old min value.
import java.util.*;
class Main
{
public static void main(String[] args)
{
Scanner sc = new Scanner(System.in);
System.out.print("Enter the size of array: ");
int size = sc.nextInt();
int arr[] = new int[size];
for(int i=0; i<size; i++) {
System.out.print("Please give value for index "+ i +" : ");
arr[i] = sc.nextInt();
}
int max = arr[0];
int min = arr[0];
for (int i = 1; i < arr.length; i++)
{
if (arr[i] > max) {
max = arr[i];
}
else if (arr[i] < min) {
min = arr[i];
}
}
System.out.println("Minimum array element is " + min);
System.out.println("Maximum array element is " + max);
}
}
Output
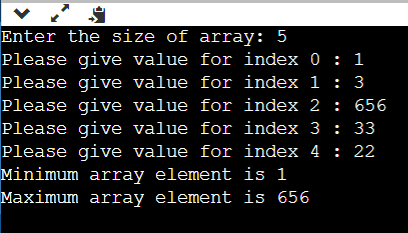