In this tutorial, you are going to learn to write Java Program to find the second largest element in an array. To Find the second largest or we can say the second maximum number in an array, first we will take the array input from the users.
We will see both types of examples where we will take the array from the users as well as the fixed array.
Example:
1). Suppose we have an array arr = [23, 11, 34, 21, 56, 36]
The second maximum number in the above array is 36
So the output will be 36
2). Suppose we have an array arr = [34, 21, 56, 56]
The second maximum number in the above array is 34
So the output will be 34
Program 1: Brute force approach to find the second largest element in the array
- This program is very simple where we will be following the brute force approach to find the second largest element in the array.
- Here we will be sorting the array in ascending order.
- To find the second largest or maximum element we will be selecting an element that is not equal to the largest element from the sorted array.
import java.util.*;
public class Main
{
public static void main(String[] args) {
int arr[] = { 11, 45, 10, 10, 32, 76};
int n = arr.length;
Arrays.sort(arr);
int secondLargest = 0;
for (int i = n - 2; i >= 0; i--)
{
if (arr[i] != arr[n - 1])
{
secondLargest = arr[i];
break;
}
}
System.out.println("Second largest/maximum number is: " + secondLargest);
}
}
Output
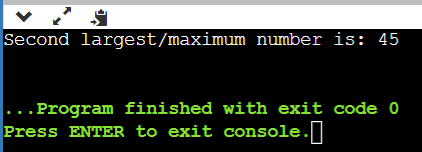
Program 2: Brute force to find the second largest in the array, user input array
- This program is very similar to the above program.
- Only difference is here we have taken dynamic array, Means at run time we are asking the array size and input elements from the user.
import java.util.*;
public class Main
{
public static void main(String[] args) {
int secondLargest = 0;
Scanner sc = new Scanner(System.in);
System.out.print("Enter the size of array: ");
int size = sc.nextInt();
int arr[] = new int[size];
for(int i=0; i<size; i++) {
System.out.print("Please give value for index "+ i +" : ");
arr[i] = sc.nextInt();
}
Arrays.sort(arr);
for (int i = size - 2; i >= 0; i--)
{
if (arr[i] != arr[size - 1])
{
secondLargest = arr[i];
break;
}
}
System.out.println("Second largest/maximum number is: " + secondLargest);
}
}
Output
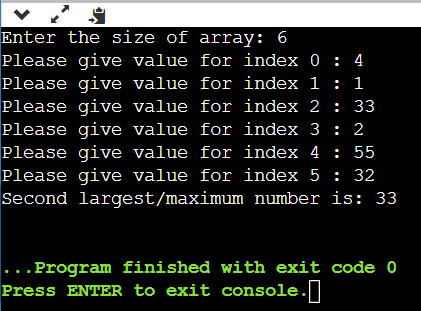
Program 3: Find Second Largest using Math.Max() in Java
- In this approach to find the second largest elements in the array, we are first finding the largest element in the array using for loop and Math.max() method.
- Now after finding the largest then using the same logic we are finding the second largest only the condition is, that should not equal to the largest.
import java.util.*;
public class Main
{
public static void main(String[] args) {
int i, largest, secondLargest;
int arr[] = { 21, 30, 11, 9, 44, 41 };
int arrSize = arr.length;
if (arrSize < 2)
{
System.out.println(" Input is Invalid ");
return;
}
largest = secondLargest = Integer.MIN_VALUE;
for(i = 0; i < arrSize; i++)
{
largest = Math.max(largest, arr[i]);
}
for(i = 0; i < arrSize; i++)
{
if (arr[i] != largest)
secondLargest = Math.max(secondLargest, arr[i]);
}
System.out.println("Second largest element is : "+ secondLargest);
}
}
Output
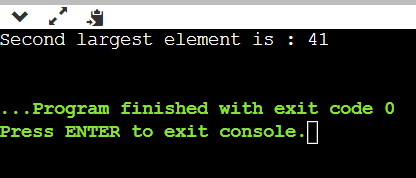
Program 4: Own logic to find second largest in array using Java
- In this program we have build own logic to find the second largest element in the array.
- We have taken the two variables largest and secondLargest.
- After comparison each array elements we will be finding the largest element.
- And to find second largest we are checking another largest which is not a largest.
import java.util.*;
public class Main
{
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("Enter the size of array: ");
int arrSize = sc.nextInt();
int arr[] = new int[arrSize];
int largest, secondLargest;
largest = secondLargest = Integer.MIN_VALUE;
System.out.println("Enter " +arrSize+ " array elements ");
for(int i=0; i<arrSize; i++) {
arr[i] = sc.nextInt();
}
if (arrSize < 2)
{
System.out.println(" Input is Invalid ");
return;
}
for (int i = 0; i < arrSize; i++) {
if (arr[i] > largest) {
secondLargest = largest;
largest = arr[i];
}
else if (arr[i] > secondLargest && arr[i] != largest)
secondLargest = arr[i];
}
System.out.println("Second largest element is : "+ secondLargest);
}
}
Output
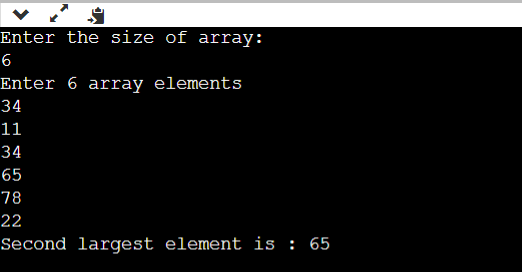