In this tutorial, we will be learning the writing a java program to print all negative numbers present in an array. To check the negative number in java there are various ways. In this program, we will be learning two types of programs.
The first way to check negative numbers our logic will be, using the if else statement we check if the number is smaller than zero.
The second way to check a negative number is by using signum() function which is available in the Math package.
Program 1 : Print all negative numbers in an array using signum() Java
- In the below program, we are using the signum() function of the Math package.
- This method is taking the double as an input and will return -1.0 if the number is negative.
- So using the if statement we will check if signum() returning -1.0 or not.
- If returning -1.0 then the condition will satisfy and will print the negative number as an output.
import java.util.*;
import java.lang.Math.*;
class Main{
public static void main(String ...a){
Scanner sc = new Scanner(System.in);
System.out.println("Enter the size of array: ");
int n = sc.nextInt();
Double arr[] = new Double[n];
int negativeCount=0;
System.out.println("Enter " +(n)+ " array elements: ");
for(int i=0; i<n; i++) {
arr[i] = sc.nextDouble();
}
System.out.println("Negative numbers are : ");
for(int i=0; i<arr.length; i++){
if(Math.signum(arr[i]) == -1.0){
System.out.println(Math.round(arr[i]));
}
}
}
}
Output
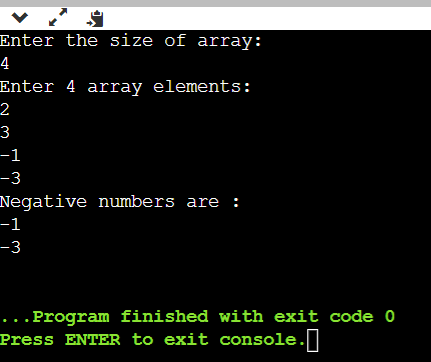
Program 2 : Java Program to Print all negative numbers in an array
- In the below program, using if else conditions we are checking array element is smaller than zero or not.
- If the array element is smaller than zero, then print the value which will be negative.
- If the array element is not smaller than zero then skip that number.
import java.util.*;
import java.lang.Math.*;
class Main{
public static void main(String ...a){
Scanner sc = new Scanner(System.in);
System.out.println("Enter the size of array: ");
int n = sc.nextInt();
Double arr[] = new Double[n];
int negativeCount=0;
System.out.println("Enter " +(n)+ " array elements: ");
for(int i=0; i<n; i++) {
arr[i] = sc.nextDouble();
}
System.out.println("Negative numbers are : ");
for(int i=0; i<arr.length; i++){
if(arr[i] < 0){
System.out.println(Math.round(arr[i]));
}
}
}
}
Output
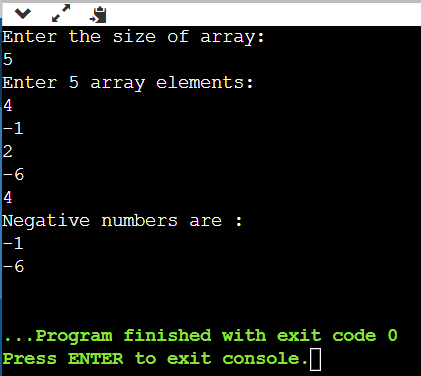