Dynamic method dispatch is also known as runtime polymorphism. Here at the run time JVM resolve of which class overridden method is called. When we call an overridden method by a reference, java determines which version for that method will be executed based on which type of object refers. It means if the object is of child class then the method should be called the child class.
Basically here upcasting concept of java is followed to achieve dynamic method dispatch.
We can define upcasting as when the reference variable of parent class refers to the object of Child class this is known as Upcasting.
See small example :
Parent p=new Parent();
Child c=new Child();
p=new Child();
So here using the reference variable of Parent class at runtime we can make an object of Child class and can call overridden method of the child class.
Lets see an example of dynamic method dispatch
class Rectangle
{
int l=2,b=3;
int area;
public void area()
{
area=l*b;
System.out.println(“Rectangle area= “+area);
}
}
class Triangle extends Rectangle
{
public void area()
{
area=(b*l)/2;
System.out.println(“Triangle area = “+area);
}
}
class Square extends Rectangle
{
public void area()
{
area=l*l;
System.out.println(“Square area= “+area);
}
}
public class Main
{
public static void main(String args[])
{
Rectangle r=new Rectangle();
r.area();
r=new Triangle();
r.area();
r=new Square();
r.area();
}
}
Output:
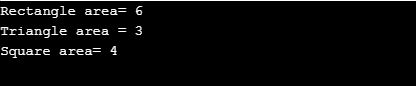
In the above example for dynamic method dispatch, you can see that we have one parent class Rectangle and two child class Triangle and Square which have extended Rectangle class. Also, the method area() is overridden by both Triangle and Rectangle Class.
So In the Main class reference variable is of Rectangle class and at the runtime it is decided which area method will be call on the basis of object of which class is created.
Advantage of dynamic method dispatch
Dynamic method dispatch allows Java to support the overriding of methods.
This overriding of the method is core for the runtime polymorphism.