In this Java Program tutorial, we will learn writing program to print a deck of cards. We all know that there are 52 cards in the deck. And Each of the card has some value and each cards belongs to one of the four suits.
So today in this java programming tutorial we are going to do exact similar thing like will create 52 cards and assign it to their belongs with value.
Java Program to Print the Deck of cards
[java]public class Main {
public static void main(String[] args) {
String[] card_number = new String[13];
for(int i=1;i<card_number.length;i++){
if(i==1){
card_number[i]=”Ace”;
}else if(i==11){
card_number[i]=”Jack”;
}else if(i==12){
card_number[i]=”Queen”;
}else if(i==13){
card_number[i]=”King”;
}else{
card_number[i]=Integer.toString(i);
}
}
String[] card_suit = {“Clubs”, “Hearts”, “Spades”, “Diamonds”};
int len = 52;
String[] cards_deck = new String[len];
int suit_len=card_suit.length;
for (int i=0; i<card_number.length; i++) {
for (int j=0; j<suit_len; j++) {
cards_deck[(suit_len)*i + j] = card_number[i] + ” of ” + card_suit[j];
}
}
for (int i=0; i<len; i++) {
int index = i + (int) (Math.random() * (len-i));
String temp = cards_deck[index];
cards_deck[index] = cards_deck[i];
cards_deck[i] = temp;
}
for (int i=0; i<len; i++) {
System.out.println(cards_deck[i]);
}
}
}
[/java]
Output
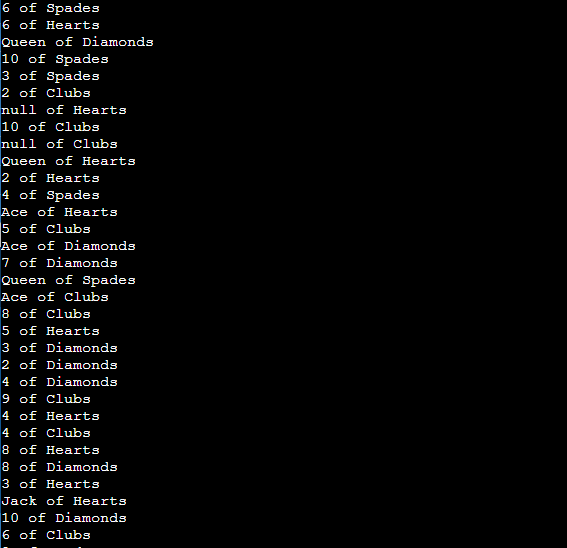
Explanation of Print the Deck of cards
In this program, we will first define two arrays. One array for deck suits and second array for deck numbers. We all know that the length of deck is 52 so we will define another array of size 52 to represent our deck size.
After that, we will initialize our deck with default values by iterating over number and suits in a nested loop.
While initializing the array, have a look at the index carefully. I have used a single dimension array for the deck here. To ensure that all the ranks are stored sequentially, I have multiplied i by the length of the suits array and added j for assignment of each suit.